Building Python Programs
Auteurs : Reges Stuart, Stepp Marty, Obourn Allison
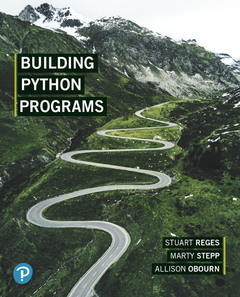
For courses in Java programming.
A layered, back-to-basics approach to Python programming
The authors of the long successful title, Building Java Programs, bring their proven and class-tested, back-to-basics strategy to teaching Python programming for the first time in Building Python Programs. Their signature layered approach introduces programming fundamentals first, with new syntax and concepts added over multiple chapters. Object-oriented programming is discussed only after students have developed a basic understanding of Python programming. This newly published textfocuses on problem solving with an emphasis on algorithmic thinking and is appropriate for the two-semester sequence in introductory computer science.
Also available with MyLab Programming
By combining trusted author content with digital tools and a flexible platform, MyLab personalizes the learning experience and improves results for each student.With MyLab Programming, students work through hundreds of short, auto-graded coding exercises and receive immediate and helpful feedback based on their work.
Note: You are purchasing a standalone product; MyLab Programming does not come packaged with this content. Students, if interested in purchasing this title with MyLab Programming, ask your instructor for the correct package ISBN and Course ID. Instructors, contact your Pearson representative for more information.
If you would like to purchase both the physical text and MyLab Programming, search for:
0135287057/9780135287057 Building Python Programs Plus MyLab Programming with Pearson eText -- Access Card Package
Package consists of:
- 0135201276 / 9780135201275 MyLab Programming with Pearson eText -- Access Card -- for Building Python Programs
- 0135205980 / 9780135205983 Building Python Programs
Table of Contents
Chapter 1 Introduction to Python Programming
- 1.1 Basic Computing Concepts
- Why Programming?
- Hardware and Software
- The Digital Realm
- The Process of Programming
- Why Python?
- The Python Programming Environment
- 1.2 And Now: Python
- Printing Output
- String Literals (Strings)
- Escape Sequences
- Printing a Complex Figure
- Comments, Whitespace, and Readability
- 1.3 Program Errors
- Syntax Errors
- Logic Errors (Bugs)
- 1.4 Procedural Decomposition
- Functions
- Flow of Control
- Identifiers and Keywords
- Functions That Call Other Functions
- An Example Runtime Error
- 1.5 Case Study: Drawing Figures
- Structured Version
- Final Version without Redundancy
- Analysis of Flow of Execution
Chapter 2 Data and Definite Loops
- 2.1 Basic Data Concepts
- Types
- Expressions
- Literals
- Arithmetic Operators
- Precedence
- Mixing and Converting Types
- 2.2 Variables
- A Program with Variables
- Increment/Decrement Operators
- Printing Multiple Values
- 2.3 The for Loop
- Using a Loop Variable
- Details about Ranges
- String Multiplication and Printing Partial Lines
- Nested for Loops
- 2.4 Managing Complexity
- Scope
- Pseudocode
- Constants
- 2.5 Case Study: Hourglass Figure
- Problem Decomposition and Pseudocode
- Initial Structured Version
- Adding a Constant
Chapter 3 Parameters and Graphics
- 3.1 Parameters
- The Mechanics of Parameters
- Limitations of Parameters
- Multiple Parameters
- Parameters versus Constants
- Optional Parameters
- 3.2 Returning Values
- The math Module
- The random Module
- Defining Functions That Return Values
- Returning Multiple Values
- 3.3 Interactive Programs
- Sample Interactive Program
- 3.4 Graphics
- Introduction to DrawingPanel
- Drawing Lines and Shapes
- Colors
- Drawing with Loops
- Text and Fonts
- Images
- Procedural Decomposition with Graphics
- 3.5 Case Study: Projectile Trajectory
- Unstructured Solution
- Structured Solution
- Graphical Version
Chapter 4 Conditional Execution
- 4.1 if/else Statements
- Relational Operators
- Nested if/else Statements
- Factoring if/else Statements
- Testing Multiple Conditions
- 4.2 Cumulative Algorithms
- Cumulative Sum
- Min/Max Loops
- Cumulative Sum with if
- Roundoff Errors
- 4.3 Functions with Conditional Execution
- Preconditions and Postconditions
- Raising Exceptions
- Revisiting Return Values
- Reasoning about Paths
- 4.4 Strings
- String Methods
- Accessing Characters by Index
- Converting between Letters and Numbers
- Cumulative Text Algorithms
- 4.5 Case Study: Basal Metabolic Rate
- One-Person Unstructured Solution
- Two-Person Unstructured Solution
- Two-Person Structured Solution
- Procedural Design Heuristics
Chapter 5 Program Logic and Indefinite Loops
- 5.1 The while Loop
- A Loop to Find the Smallest Divisor
- Loop Priming
- 5.2 Fencepost Algorithms
- Fencepost with if
- Sentinel Loops
- Sentinel with Min/Max
- 5.3 Boolean Logic
- Logical Operators
- Boolean Variables and Flags
- Predicate Functions
- Boolean Zen
- Short-Circuited Evaluation
- 5.4 Robust Programs
- The try/except Statement
- Handling User Errors
- 5.5 Assertions and Program Logic
- Reasoning about Assertions
- A Detailed Assertions Example
- 5.6 Case Study: Number Guessing Game
- Initial Version without Hinting
- Randomized Version with Hinting
- Final Robust Version
Chapter 6 File Processing
- 6.1 File-Reading Basics
- Data and Files
- Reading a File in Python
- Line-Based File Processing
- Structure of Files and Consuming Input
- Prompting for a File
- 6.2 Token-Based Processing
- Numeric Input
- Handling Invalid Input
- Mixing Lines and Tokens
- Handling Varying Numbers of Tokens
- Complex Input Files
- 6.3 Advanced File Processing
- Multi-Line Input Records
- File Output
- Reading Data from the Web
- 6.4 Case Study: ZIP Code Lookup
Chapter 7 Lists
- 7.1 List Basics
- Creating Lists
- Accessing List Elements
- Traversing a List
- A Complete List Program
- Random Access
- List Methods
- 7.2 List-Traversal Algorithms
- Lists as Parameters
- Searching a List
- Replacing and Removing Values
- Reversing a List
- Shifting Values in a List
- Nested Loop Algorithms
- List Comprehensions
- 7.3 Reference Semantics
- Values and References
- Modifying a List Parameter
- The Value None
- Mutability
- Tuples
- 7.4 Multidimensional Lists
- Rectangular Lists
- Jagged Lists
- Lists of Pixels
- 7.5 Case Study: Benford’s Law
- Tallying Values
- Completing the Program
Chapter 8 Dictionaries and Sets
- 8.1 Dictionary Basics
- Creating a Dictionary
- Dictionary Operations
- Looping Over a Dictionary
- Dictionary Ordering
- 8.2 Advanced Dictionary Usage
- Dictionary for Tallying
- Nested Collections
- Dictionary Comprehensions
- 8.3 Sets
- Set Basics
- Set Operations
- Set Efficiency
- Set Example: Lottery
Chapter 9 Recursion
- 9.1 Thinking Recursively
- A Nonprogramming Example
- Iteration to Recursion
- Structure of Recursive Solutions
- Reversing a File
- The Recursive Call Stack
- 9.2 Recursive Functions and Data
- Integer Exponentiation
- Greatest Common Divisor
- Directory Crawler
- 9.3 Recursive Graphics
- Cantor Set
- Sierpinski Triangle
- 9.4 Recursive Backtracking
- Traveling North/East
- Eight Queens Puzzle
- Stopping after One Solution
- 9.5 Case Study: Prefix Evaluator
- Infix, Prefix, and Postfix Notation
- Evaluating Prefix Expressions
- Complete Program
Chapter 10 Searching and Sorting
- 10.1 Searching and Sorting Libraries
- Binary Search
About our authors
Stuart Reges is a Principal Lecturer in the Paul G. Allen School of Computer Science and Engineering at the University of Washington. He manages the introductory programming classes, participates in the design of undergraduate curricula, and is involved in K to 12 outreach. He held similar positions at Stanford University and the University of Arizona in a career spanning 30 years. In 1985 he won the Dinkelspiel Award for Outstanding Service to Undergraduate Education at Stanford. In 2012 he won the Distinguished Teaching Award, which is the highest award given by UW for teaching.
Allison Obourn holds a position as Senior Lecturer in the Computer Science Department at the University of Arizona. She worked as a Lecturer at the University of Washington Computer Science & Engineering Department prior to moving to the University of Arizona. At both schools, she has specialized in teaching introductory programming and web programming. She holds a master’s degree from the University of Washington Computer Science & Engineering Department.
Date de parution : 08-2018
Ouvrage de 832 p.
10x10 cm